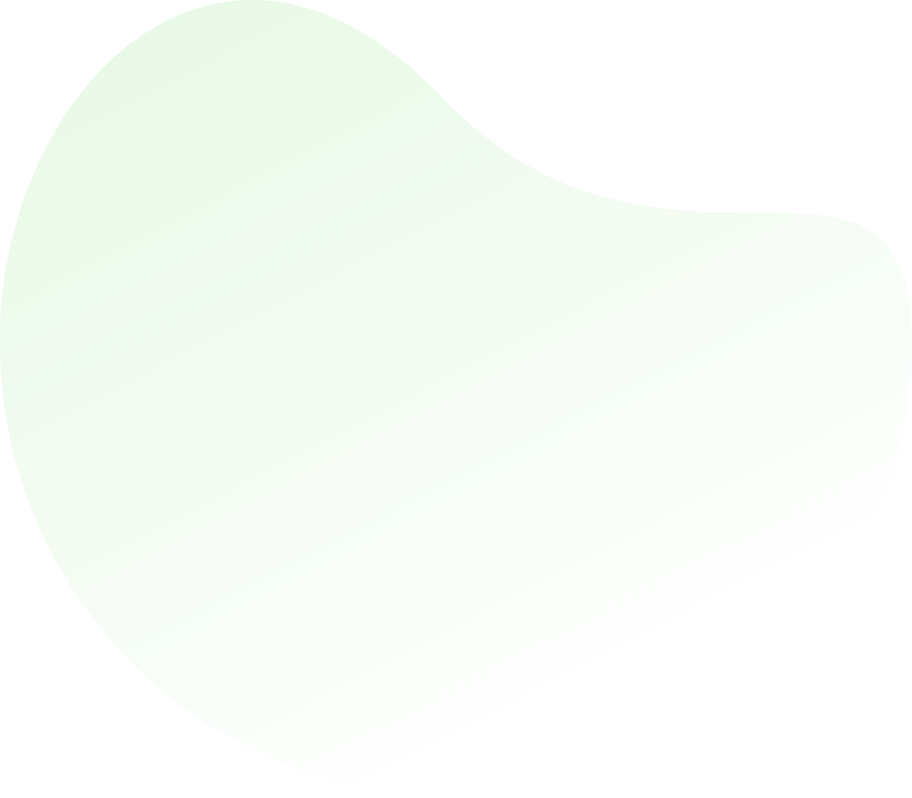
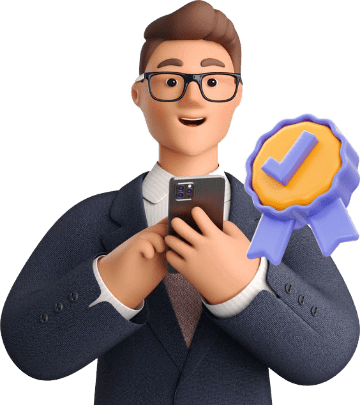
For help Students Orientation
Mcqs Questions
One stop destination for examination, preparation, recruitment, and more. Specially designed online test to solve all your preparation worries. Go wherever you want to and practice whenever you want, using the online test platform.